Google Maps Autocomplete Address is a powerful feature that enhances user experience by providing real-time address suggestions as users type. This functionality, powered by Google’s Places API, helps ensure accurate address input while saving time. In this tutorial, we’ll walk through integrating this feature into a Laravel 12 application.
Understanding Google Maps Autocomplete
The Google Maps Autocomplete feature works by:
- Predicting address suggestions as users type in the search field
- Displaying these suggestions in a dropdown menu
- Providing complete address details including latitude and longitude coordinates
- Reducing input errors and improving data accuracy
Implementation Steps
Step 1: Set Up the Route
First, we’ll create a route in routes/web.php:
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\GoogleController;
Route::get('google-autocomplete', [GoogleController::class, 'index']);
Step 2: Create the Controller
Next, generate a controller to handle our view:
<?php
namespace App\Http\Controllers;
use Illuminate\View\View;
class GoogleController extends Controller
{
/**
* Display the Google Autocomplete form
*
* @return View
*/
public function index(): View
{
return view('googleAutocomplete');
}
}
Step 3: Configure Google Maps API Key
Add your Google Maps API key to your .env file:
GOOGLE_MAP_KEY=your_google_api_key_here
Step 4: Create the Blade View
Create a view file at resources/views/googleAutocomplete.blade.php:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Google Autocomplete Address in Laravel 12</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="container py-5">
<div class="card shadow">
<div class="card-header bg-primary text-white">
<h3 class="mb-0">Google Autocomplete Address Integration</h3>
</div>
<div class="card-body">
<form id="addressForm">
<div class="mb-3">
<label for="autocomplete" class="form-label">Start typing your address</label>
<input type="text" class="form-control" id="autocomplete"
placeholder="Enter street, city, or zip code" autocomplete="off">
</div>
<div class="row mb-3 d-none" id="coordinatesSection">
<div class="col-md-6">
<label for="latitude" class="form-label">Latitude</label>
<input type="text" class="form-control" id="latitude" readonly>
</div>
<div class="col-md-6">
<label for="longitude" class="form-label">Longitude</label>
<input type="text" class="form-control" id="longitude" readonly>
</div>
</div>
<button type="submit" class="btn btn-primary">Submit Address</button>
</form>
</div>
</div>
</div>
<!-- JavaScript Libraries -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
<!-- Google Maps API -->
<script src="https://maps.googleapis.com/maps/api/js?key={{ env('GOOGLE_MAP_KEY') }}&libraries=places"></script>
<!-- Custom Script -->
<script>
$(document).ready(function() {
// Initialize Google Places Autocomplete
function initAutocomplete() {
const input = document.getElementById('autocomplete');
const autocomplete = new google.maps.places.Autocomplete(input);
autocomplete.addListener('place_changed', function() {
const place = autocomplete.getPlace();
if (!place.geometry) {
alert("No details available for input: '" + place.name + "'");
return;
}
// Update latitude and longitude fields
$('#latitude').val(place.geometry.location.lat());
$('#longitude').val(place.geometry.location.lng());
// Show coordinates section
$('#coordinatesSection').removeClass('d-none');
});
}
// Load the autocomplete functionality
google.maps.event.addDomListener(window, 'load', initAutocomplete);
// Handle form submission
$('#addressForm').on('submit', function(e) {
e.preventDefault();
alert('Address submitted successfully!');
// Add your form submission logic here
});
});
</script>
</body>
</html>
Step 5: Run the Application
Start your Laravel development server:
php artisan serve
Visit the application in your browser at:
http://localhost:8000/google-autocomplete
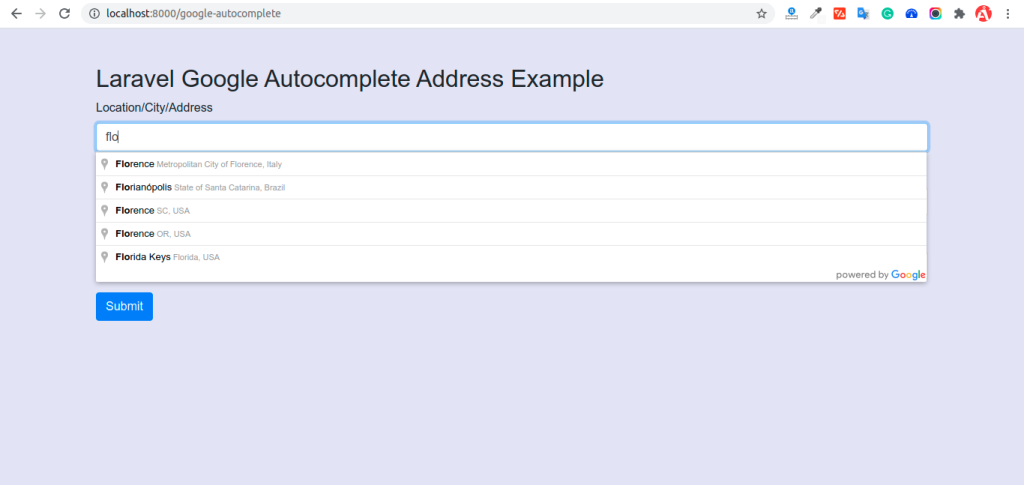