In today’s digital world, automating document generation and email delivery is crucial for many web applications. Whether you’re sending invoices, reports, or other important documents, Laravel makes this process remarkably simple. In this tutorial, I’ll walk you through how to generate PDF files and send them as email attachments in a Laravel 12 application.
Why This Combination Matters
PDF generation and email delivery are two powerful features that, when combined, can automate many business processes. Laravel’s ecosystem provides excellent packages for both functionalities, allowing developers to implement these features with minimal code.
For our example, we’ll use:
- dompdf for PDF generation
- Laravel’s built-in Mail system with Gmail SMTP for delivery
Step-by-Step Implementation
1. Setting Up the Laravel Environment
First, ensure you have a fresh Laravel 12 installation. If you haven’t created your project yet, run:
composer create-project laravel/laravel example-app
2. Installing dompdf Package
Laravel doesn’t include PDF generation out of the box, but the excellent barryvdh/laravel-dompdf package makes this easy:
composer require barryvdh/laravel-dompdf
This package provides a simple interface for converting HTML views to PDF documents.
3. Configuring Email Settings
For email functionality, we need to configure our .env file with SMTP details. Here’s a Gmail configuration example:
MAIL_DRIVER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=587
MAIL_USERNAME=mygoogle@gmail.com
MAIL_PASSWORD=rrnnucvnqlbsl
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=mygoogle@gmail.com
MAIL_FROM_NAME="${APP_NAME}"
Important security note: For production, consider using app-specific passwords or a dedicated email service.
4. Creating the Mail Class
Laravel’s Mailables make email creation clean and organized. Generate a new mail class:
php artisan make:mail MailExample
Here’s how we’ll structure our MailExample.php:
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Mail\Mailables\Content;
use Illuminate\Mail\Mailables\Envelope;
use Illuminate\Queue\SerializesModels;
use Illuminate\Mail\Mailables\Attachment;
class MailExample extends Mailable
{
use Queueable, SerializesModels;
public $mailData;
public function __construct($mailData)
{
$this->mailData = $mailData;
}
public function envelope(): Envelope
{
return new Envelope(
subject: $this->mailData['title'],
);
}
public function content(): Content
{
return new Content(
view: 'emails.myTestMail',
with: $this->mailData
);
}
public function attachments(): array
{
return [
Attachment::fromData(
fn () => $this->mailData['pdf']->output(),
'Report.pdf'
)->withMime('application/pdf'),
];
}
}
5. Setting Up Routes
Add a simple route to trigger our PDF generation and email sending:
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PDFController;
Route::get('send-email-pdf', [PDFController::class, 'index']);
6. Creating the Controller
Our PDFController will handle the logic:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Mail\MailExample;
use PDF;
use Mail;
class PDFController extends Controller
{
public function index()
{
$data["email"] = "your@gmail.com";
$data["title"] = "From ItSolutionStuff.com";
$data["body"] = "This is Demo";
$pdf = PDF::loadView('emails.myTestMail', $data);
$data["pdf"] = $pdf;
Mail::to($data["email"])->send(new MailExample($data));
dd('Mail sent successfully');
}
}
7. Designing the PDF Template
Create a Blade template at resources/views/emails/myTestMail.blade.php:
<!DOCTYPE html>
<html>
<head>
<title>ERPlytics.com/</title>
</head>
<body>
<h1>{{ $title }}</h1>
<p>{{ $body }}</p>
<p>Thank you</p>
</body>
</html>
This template serves both as the email content and the PDF content.
Running the Application
Start your Laravel development server:
php artisan serve
Visit http://localhost:8000/send-email-pdf in your browser to trigger the process. You should see a “Mail sent successfully” message, and the email with PDF attachment should arrive in the recipient’s inbox.
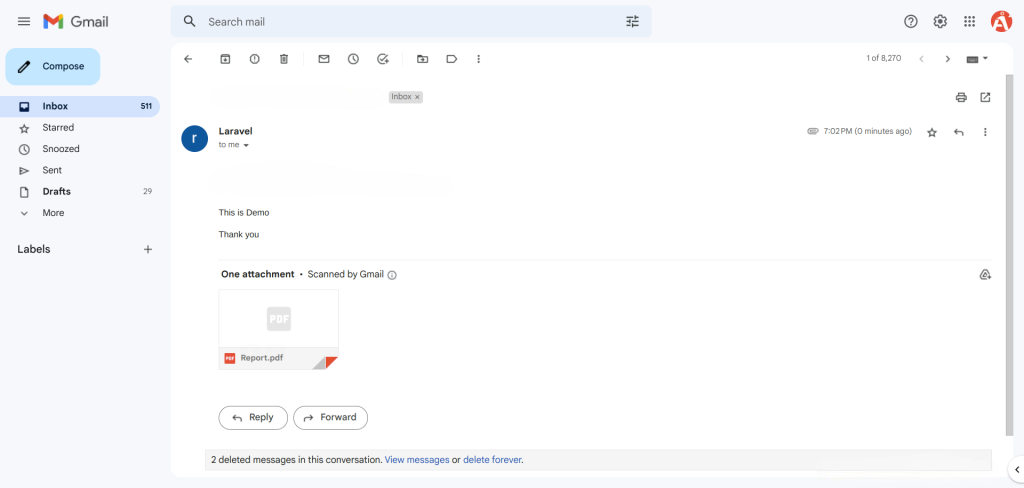